Overview : 프린트시 백그라운드 컬러 나오게 하기
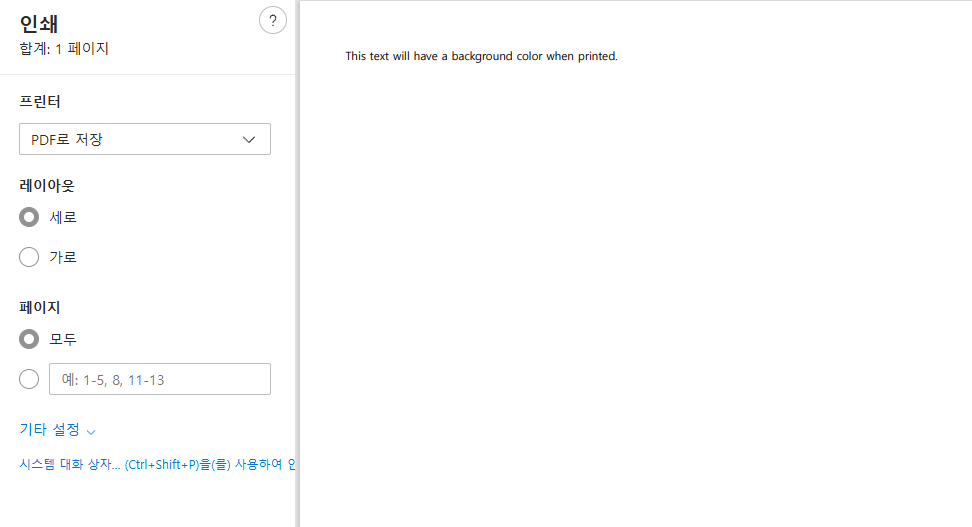
프린트할 때 배경색 유지하기
1 2 3 4 5 6 7 8 9 10 |
<style> @media print { .print-style { -webkit-print-color-adjust: exact; /* Chrome, Safari 등 웹킷 기반 브라우저용 */ print-color-adjust: exact; /* 표준 속성 */ } } </style> |
대부분의 브라우저는 기본적으로 프린트 시 배경색을 출력하지 않도록 설정되어 있습니다. 이를 해결하기 위해, @media print
규칙을 사용하여 프린트할 때 배경색이 출력되도록 설정할 수 있습니다.
html + css
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Print Background Example</title> <style> @media print { .print-style { -webkit-print-color-adjust: exact; /* For webkit-based browsers like Chrome and Safari */ print-color-adjust: exact; /* Standard property */ } } </style> </head> <body> <div class="print-style" style="background-color: yellow; color: black; padding: 10px;"> This text will have a background color when printed. </div> </body> </html> |
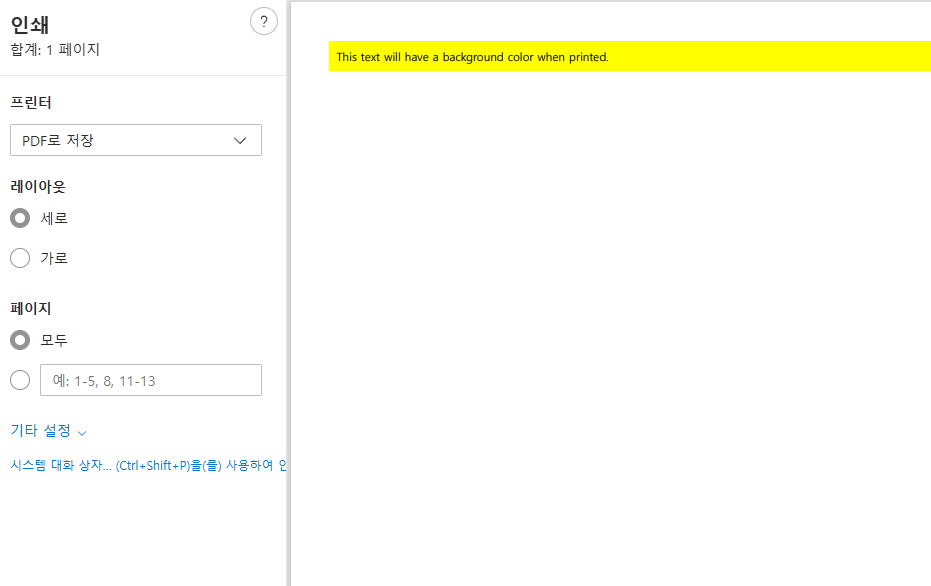
JavaScript를 사용하여 특정 div
요소만 인쇄하려면, 일반적인 접근 방식은 해당 div
의 콘텐츠를 새로운 창에 복사하고 그 창에서 인쇄를 실행하는 것입니다. 이렇게 하면 페이지의 다른 요소들은 제외하고 특정 div
만 출력할 수 있습니다. 아래는 이를 구현하는 방법의 예입니다.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 |
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Print Specific Div</title> </head> <body> <div id="content-to-print"> <h1>This will be printed</h1> <p>This content is inside the div that will be printed.</p> </div> <div id="content-to-ignore"> <h1>This will not be printed</h1> <p>This content is outside the div and will not be printed.</p> </div> <button onclick="printDiv()">Print Specific Div</button> <script> function printDiv() { // 인쇄할 div 요소를 선택 var divToPrint = document.getElementById('content-to-print').innerHTML; // 새로운 창 열기 var newWindow = window.open('', '', 'width=800,height=600'); // 새 창에 HTML 작성 newWindow.document.write('<html><head><title>Print</title>'); newWindow.document.write('</head><body>'); newWindow.document.write(divToPrint); newWindow.document.write('</body></html>'); // 문서 닫기 및 인쇄 실행 newWindow.document.close(); newWindow.print(); } </script> </body> </html> |
배경 이미지가 인쇄되도록 하려면, 기본적으로 브라우저가 인쇄 시 배경 이미지를 제외하는 설정이 있을 수 있습니다. 하지만 JavaScript와 CSS를 사용하여 강제로 배경 이미지를 인쇄할 수 있습니다.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 |
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Print Specific Div with Background</title> <style> /* 배경 이미지를 포함한 인쇄 스타일 */ #content-to-print { background-image: url('your-background-image-url.jpg'); background-size: cover; padding: 20px; color: white; } /* 인쇄할 때 배경 이미지를 유지하도록 설정 */ @media print { #content-to-print { background-image: url('your-background-image-url.jpg') !important; -webkit-print-color-adjust: exact; /* Chrome, Safari */ print-color-adjust: exact; /* Firefox */ } } </style> </head> <body> <div id="content-to-print"> <h1>This will be printed with a background image</h1> <p>This content is inside the div that will be printed with a background image.</p> </div> <div id="content-to-ignore"> <h1>This will not be printed</h1> <p>This content is outside the div and will not be printed.</p> </div> <button onclick="printDiv()">Print Specific Div</button> <script> function printDiv() { // 인쇄할 div 요소를 선택 var divToPrint = document.getElementById('content-to-print').innerHTML; // 새로운 창 열기 var newWindow = window.open('', '', 'width=800,height=600'); // 새 창에 스타일 포함하여 HTML 작성 newWindow.document.write('<html><head><title>Print</title>'); newWindow.document.write('<style>'); newWindow.document.write('@media print {'); newWindow.document.write('#content-to-print {'); //newWindow.document.write('background-image: url("your-background-image-url.jpg") !important;'); newWindow.document.write('-webkit-print-color-adjust: exact;'); newWindow.document.write('print-color-adjust: exact;'); newWindow.document.write('}'); newWindow.document.write('}</style>'); newWindow.document.write('</head><body>'); newWindow.document.write('<div id="content-to-print">' + divToPrint + '</div>'); newWindow.document.write('</body></html>'); // 문서 닫기 및 인쇄 실행 newWindow.document.close(); newWindow.print(); } </script> </body> </html> |